안드로이드 스튜디오 이미지 슬라이더 ( ImagePager, Adapter )
2019. 12. 24. 21:01ㆍ2020/Android App Develop
< ActivityPractice.java >
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
|
package com.example.myapplication;
import android.os.Bundle;
public class PracticeActivity extends AppCompatActivity {
PracticeAdapter adapter;
ViewPager viewPager;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_practice);
viewPager = findViewById(R.id.ViewPager_Practice);
adapter = new PracticeAdapter(this);
viewPager.setAdapter(adapter);
} // OnCreate END
}
http://colorscripter.com/info#e" target="_blank" style="color:#e5e5e5text-decoration:none">Colored by Color Scripter
|
< PracticeAdapter.java >
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
|
package com.example.myapplication;
import android.content.Context;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.ImageView;
import android.widget.TextView;
import androidx.annotation.NonNull;
public class PracticeAdapter extends PagerAdapter {
private int[] images = {R.drawable.icon_happy,R.drawable.yellow_something,R.drawable.yellow_star};
private LayoutInflater inflater;
private Context context;
public PracticeAdapter(Context context){
this.context = context;
}
@Override
public int getCount() {
return images.length;
}
@Override
public boolean isViewFromObject(@NonNull View view, @NonNull Object object) {
return view==object;
}
@NonNull
@Override
public Object instantiateItem(@NonNull ViewGroup container, int position) {
inflater = (LayoutInflater) context.getSystemService(Context.LAYOUT_INFLATER_SERVICE);
View v = inflater.inflate(R.layout.activity_practice_2,container,false);
ImageView imageView = v.findViewById(R.id.ImageView_practice);
TextView textView = v.findViewById(R.id.TextView_Practice);
imageView.setImageResource(images[position]);
textView.setText((position+1) +" 번 째 이미지입니다.");
container.addView(v);
return v;
}
@Override
public void destroyItem(@NonNull ViewGroup container, int position, @NonNull Object object) {
container.invalidate();
}
}
http://colorscripter.com/info#e" target="_blank" style="color:#e5e5e5text-decoration:none">Colored by Color Scripter
|
< activity_practice.xml >
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
|
<?xml version="1.0" encoding="utf-8"?>
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".PracticeActivity">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
android:gravity="center_horizontal">
android:id="@+id/ViewPager_Practice"
android:layout_width="match_parent"
android:layout_height="match_parent"
</LinearLayout>
|
< activity_practice_2.xml > ImageView를 위한 디자인
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
|
<?xml version="1.0" encoding="utf-8"?>
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
android:gravity="center_horizontal">
<ImageView
android:id="@+id/ImageView_practice"
android:layout_width="100dp"
android:layout_height="100dp">
</ImageView>
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:textSize="30dp"
android:id="@+id/TextView_Practice">
</TextView>
</LinearLayout>
|
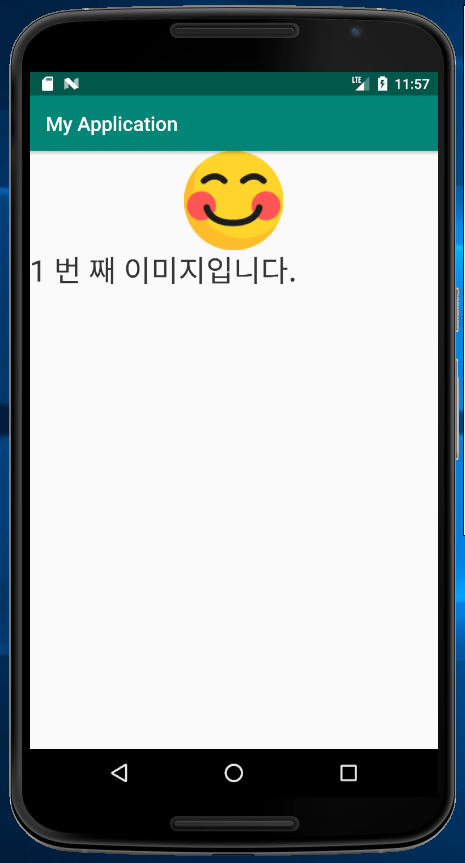
'2020 > Android App Develop' 카테고리의 다른 글
안드로이드 스튜디오 ListView, Adapter 사용법 (0) | 2019.12.24 |
---|---|
안드로이드 스튜디오 알림창 띄우기 ( AlertDIalog, Toast ) (0) | 2019.12.23 |
안드로이드 스튜디오 간단한 계산기 만들기 ( Button Click기능 ) (0) | 2019.12.23 |
안드로이드 스튜디오 뉴스앱 만들기 (NEWS API, Fresco, Volley, JSon ) (2) | 2019.12.21 |
안드로이드 스튜디오 뉴스앱 만들기 (RecyclerView) (0) | 2019.12.20 |